Understanding the Fundamentals of Design Patterns in Software
Written on
Chapter 1: Introduction to Design Patterns
"Identifying patterns is the essence of wisdom." — Dennis Prager
If you are involved in software engineering, you are likely familiar with the concept of Design Patterns. The idea of a pattern is appealing to engineers, as it signifies a consistent or typical way in which certain tasks are performed.
The concept of Design Patterns was first introduced in the late 1970s by Christopher Alexander and his colleagues. This notion was later adapted for software engineering by the "Gang of Four" (GoF) in their influential 1995 book, Design Patterns: Elements of Reusable Object-Oriented Software. The Gang of Four comprised Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides. Their ability to translate such a broad concept into the realm of software engineering was groundbreaking, and the principles outlined in their book continue to be essential knowledge for proficient software engineers.
Even after more than two decades since its publication, this book remains one of the best-sellers in software engineering, underscoring the profound impact of their work.
So, what exactly are Design Patterns? In the preface of the GoF book, there's a succinct definition: it is a collection of design patterns that offers simple and elegant solutions to specific challenges in object-oriented software design.
Contrary to popular belief, Design Patterns are not complex or mystical; they are straightforward solutions to common problems that software engineers encounter daily. They serve as abstractions for recurring issues.
One remarkable feature of Design Patterns is their independence from specific programming languages; they are focused on programming paradigms. This means that the principles discussed in the book can be applied effectively in modern object-oriented languages, ultimately leading to better solutions.
The GoF identified 23 Design Patterns, now known as the Original Design Patterns. While many other patterns existed prior to the book's publication, this article will concentrate on those foundational ones.
The Original 23 Design Patterns are categorized into three main groups based on their areas of application: Creation, Structure, and Behavior.
Section 1.1: Creational Patterns
In object-oriented programming, creating and managing objects is a routine task. A proficient programmer ensures that their code is adaptable enough to determine when, how, and where to instantiate objects automatically.
One prevalent anti-pattern in software development is the explicit creation of objects. The Creational Patterns category addresses this issue by streamlining the object creation process. The GoF outlined six Creational Patterns that assist in object creation while enhancing flexibility. These include:
- Simple Factory
- Factory Method
- Abstract Factory
- Singleton
- Builder
- Prototype
Section 1.2: Structural Patterns
A building's integrity relies on its structure, which enables it to withstand adverse conditions. The same principle applies to software systems; their robustness depends on a solid structure.
Structural Design Patterns help in constructing a resilient architecture for applications. They facilitate the organization and composition of various components, ensuring that everything is systematically arranged. The original seven Structural Design Patterns are:
- Adapter
- Bridge
- Composite
- Decorator
- Façade
- Flyweight
- Proxy
Section 1.3: Behavioral Patterns
Finally, the Behavioral Design Patterns focus on the interactions among the different entities within a system. You can think of these patterns as the gatekeepers managing the responsibilities among the objects that comprise a system. There are ten original Behavioral Design Patterns:
- Chain of Responsibility
- Command
- Interpreter
- Iterator
- Mediator
- Observer
- State
- Strategy
- Template Method
- Visitor
Having explored the three categories of Design Patterns and their original 23 types, you now possess a foundational understanding of what Design Patterns are and how the GoF categorized them.
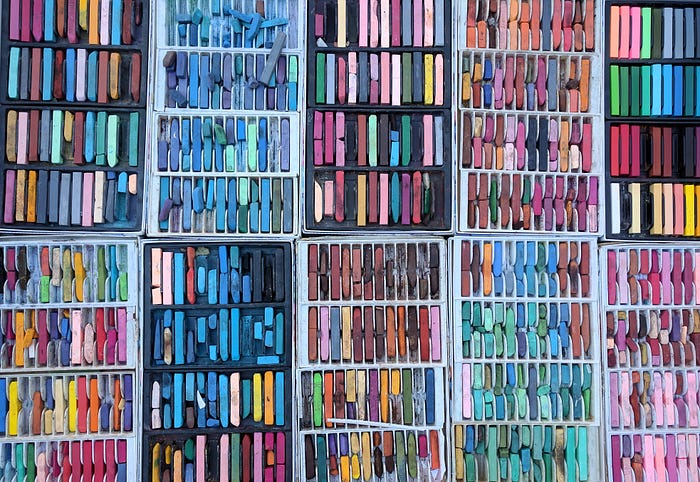
As mentioned, this article serves as an introduction to Design Patterns. Future articles will delve deeper into each category, providing detailed explanations and code implementations to illustrate how specific Design Patterns can address real-world challenges. Stay tuned for the upcoming installments in this series.
Chapter 2: Learning Resources
To further enhance your understanding of Design Patterns, here are two valuable resources:
The first video titled Design Patterns 101 | System Design | 2022 | Yogita Sharma offers an in-depth exploration of the fundamentals of design patterns, making it an excellent starting point.
The second video, What are Design Patterns and Should You Learn Them?, provides insights into the importance of design patterns and whether they are worth learning.
Nelson out!